Stripe prepared Product Purchase
The stripe-prepared product purchase function enables the use of existing SKUs from a Stripe product, allowing purchases within your flow.
Note
This method does not support
metadata
.
Prerequisites
Enabling Checkout
To use the Checkout client-only integration
, enable it in the Stripe Checkout settings.
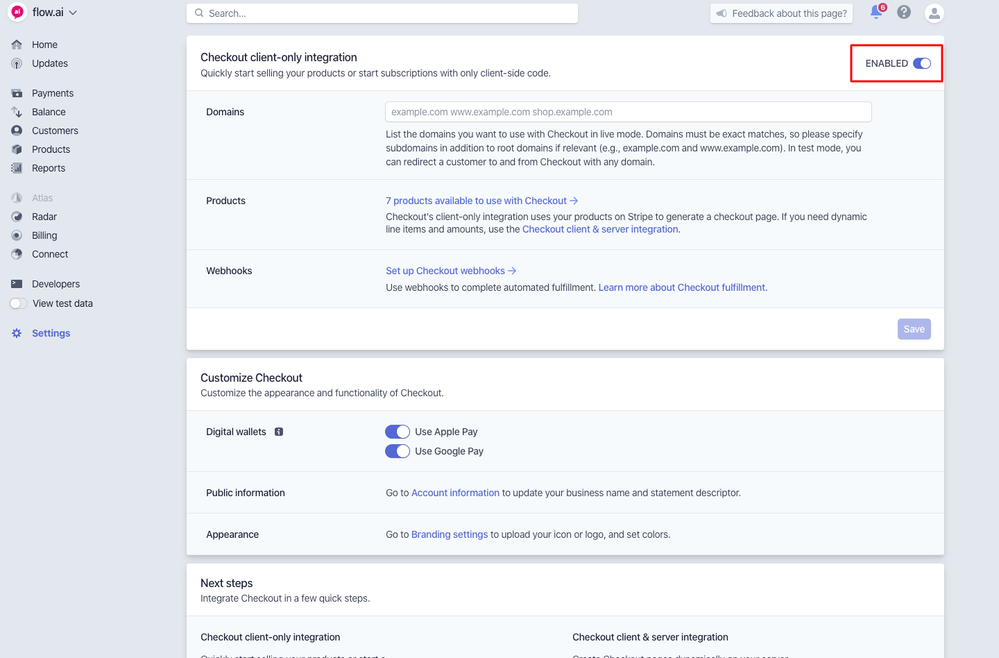
One-time purchase products
Khoros Flow supports only one-time purchase products
. When creating a new Stripe product, ensure you have selected the correct option.
Usage example
async payload => {
const url = await payments.preparedPurchase.stripe({
key: 'your_stripe_publishable_key',
locale: 'nl',
collectAddress: true,
submitType: 'donate',
items: [
{
sku: 'sku_of_your_product', quantity: 1
},
{
sku: 'sku_of_your_another_product', quantity: 3
}
],
onSuccess: {
eventName: 'bought',
params: [
new Param('category', 'clothes')
]
},
onFailure: {
eventName: 'not bought',
params: [
new Param('category', 'clothes')
]
}
})
const buttons = new Buttons('Purchase my items')
buttons.addButton(new Button({
label: 'Click to purchase',
type: 'payment',
value: url
}))
const message = new Message(`Purchase items here ${url}`)
message.addResponse(buttons)
return message
}
Properties
Property | Type | Example | Description | Required |
---|---|---|---|---|
key | string | pk_test_XXXXXXXXXXX | Your Stripe Publishable Key | Yes |
collectAddress | boolean | true | Whether Checkout should collect customer's address | No |
locale | string | nl | By default, Checkout detects the locale of the customer’s browser and displays a translated version of the page in their language if it is supported. You can also provide a specific locale for Checkout to use instead | No |
submitType | string | donate | Submit the type of payment session | No |
button.label | string | Click to purchase | Label of the confirmation button | No |
Success and Failure params
You can include onSuccess
and onFailure
configuration, that will be used in corresponding situations.
Property | Type | Example | Description | Required |
---|---|---|---|---|
eventName | string | bough | Event name to trigger when items are successfully bought / purchase is rejected | |
params | array | new Param('productiId', 112234) | Parameters that are set when the items are bought / purchase is rejected |
Items
Multiple selections are based on the number of items being purchased. Each individual item should contain sku
and quantity
properties.
Properties
Property | Type | Example | Description | Required |
---|---|---|---|---|
sku | string | sku_XXXXXXXXXXX | SKU of your product | Yes |
quantity | number | 1 | Amount of items to be purchased | Yes |
Updated 5 months ago