Replies: WhatsApp templated messages
Flow supports template messages for Cloud API business platforms. You can send templated messages using Flow reply and Code Actions, which enables you to broadcast structured information to your customers.
To send template messages, you must first integrate WhatsApp into a Flow project. Read more about WhatsApp integration and messaging capabilities.
Configure in Khoros Care
- Go to Khoros Care Configuration > WhatsApp Business Manager.
- Copy the Message Template Namespace from the WhatsApp Business Account details.
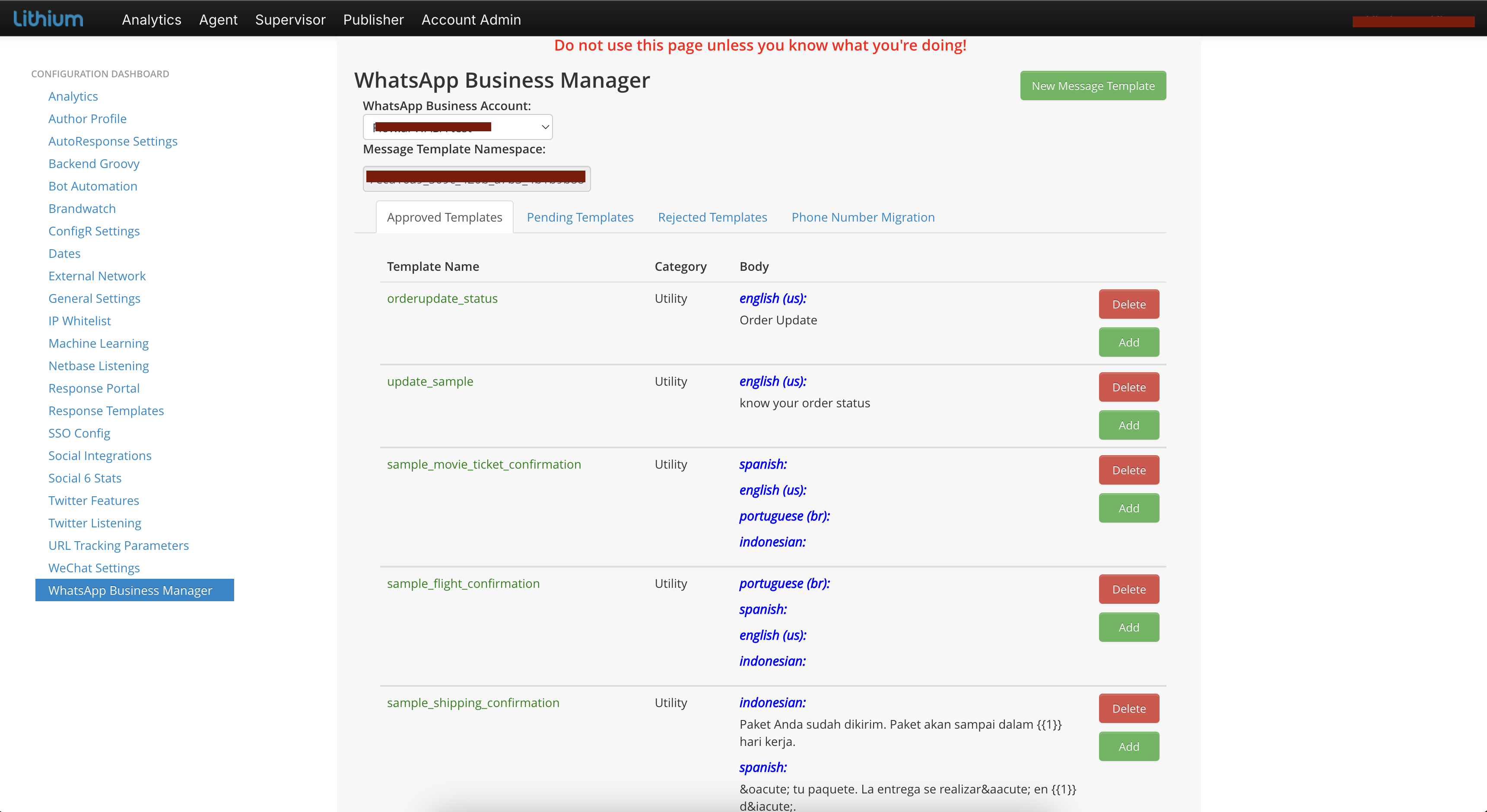
You can select any of the templates from the Approved Templates list. You need the Template namespace for the reply in the flow design.
You can implement message templates by using the Template reply or Code Actions.
Use a Template Reply
- Create a flow.
- Drag and drop the Event trigger into the canvas.
Note: We recommend starting the flow with the Event trigger to broadcast the templated message. - Drag and drop the Template reply into the canvas.
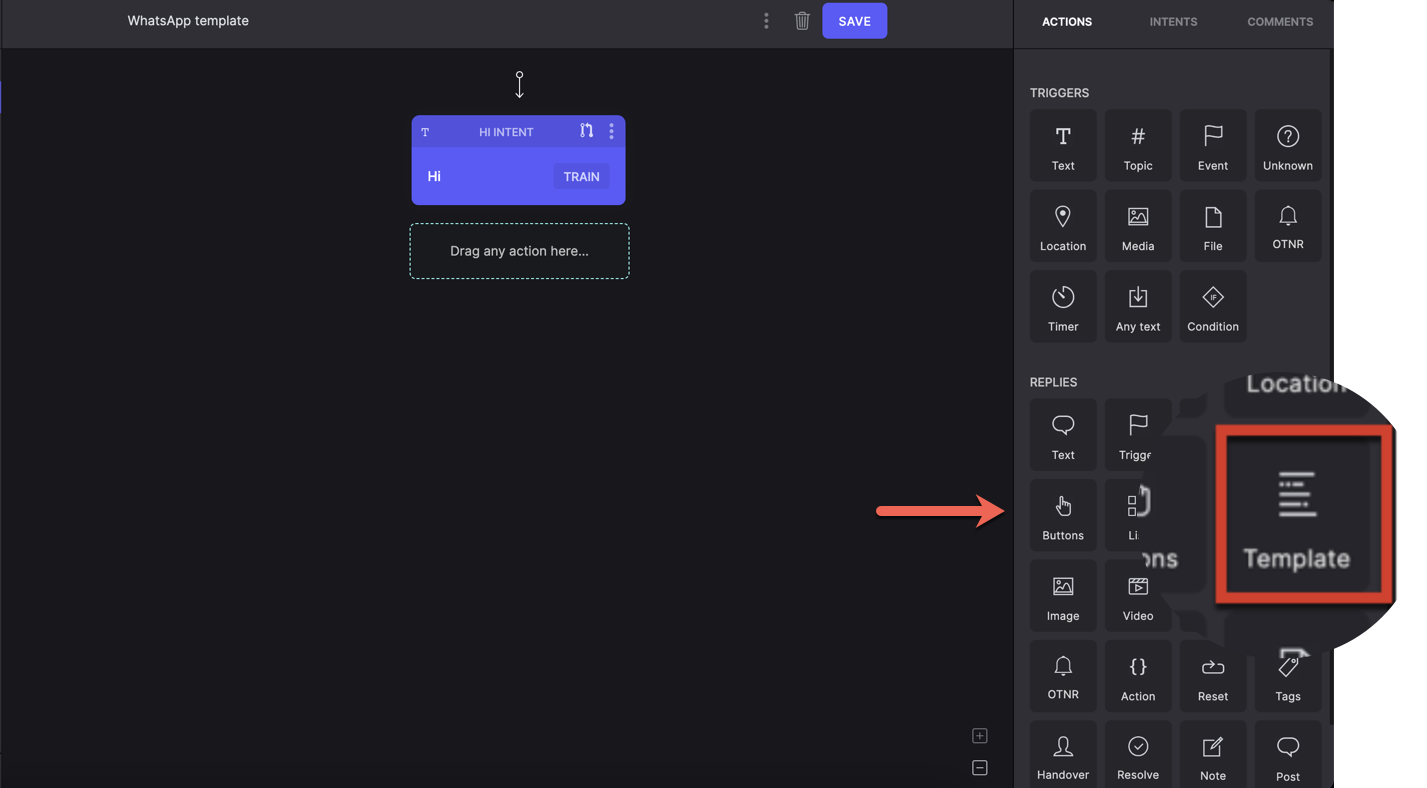
- Enter the template name in the Template field. Provide the same name as you did in Care.
- Paste the template namespace in the Namespace field.
- Choose a language from the Language drop-down menu. Select the same language you set for the template in Care.
- (Optional) Enter the template message in the Template message field. This is automatically applied based on the approved template.
Note: The entries for the above fields must be pre-approved by Whatsapp and should be as per the configurations of the selected and approved templates. - (Optional) Select a Media Header: Text, Image, Video, or File to include media in the template message. None is selected by default.
- Add all body text (if applicable) and configure it. You can use parameters in body text like regular replies {{param_name}}.
Note: If the body text is missing or invalid, your message will not be sent. - Select the Body Text type based on the message: Text, Currency, or Date.
- Enter the value in the Param field. For currency, select Currency Type and Amount. For date, select the Date and Time for a particular timezone.
- Click SAVE to save the flow.
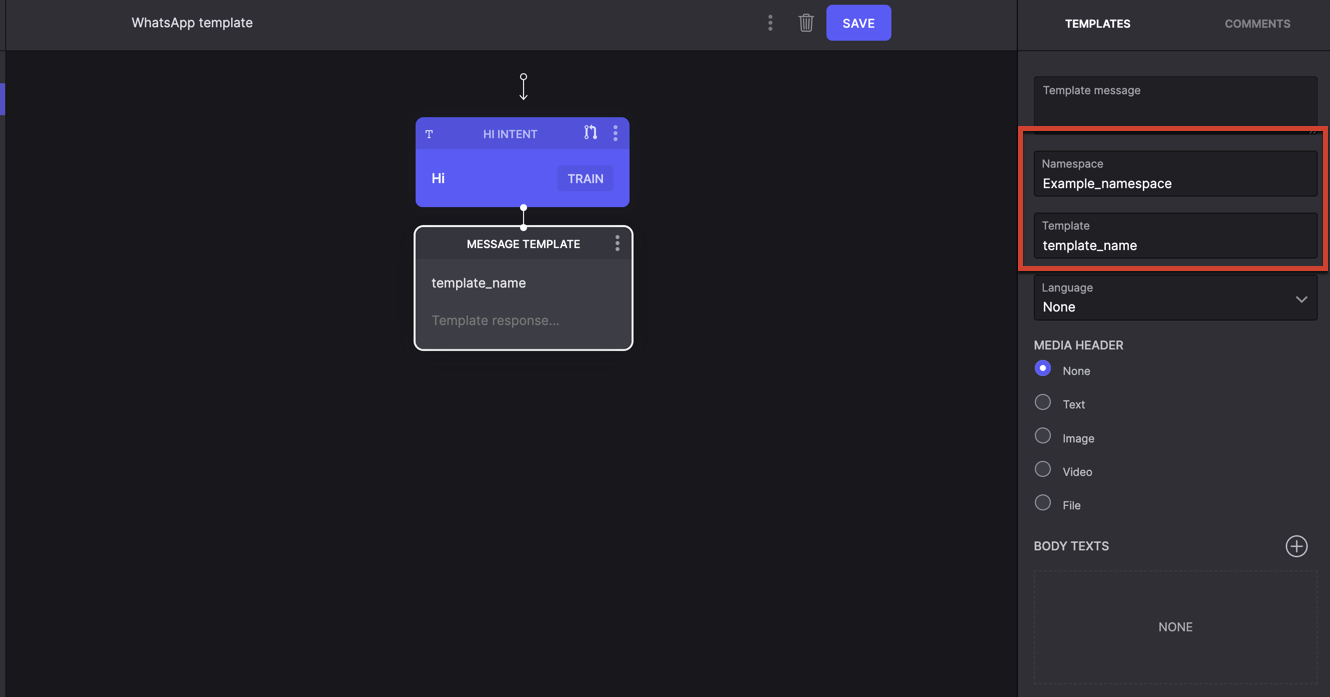
Code Action Example
You can alternatively use the code action to send templated messages on WhatsApp—the following code samples can be used for sending templated messages. Ensure that you change the variables as per your organization settings.
- Create a basic template without body text:
async payload => {
try {
const language = new WhatsApp.Language({
policy: "deterministic",
code: "en_US", // change this to supported language
})
const template = new WhatsApp.Template({
namespace: "your_namespace",
name: "your_template_name",
language: language,
components: []
})
const message = new Message("my fallback").addResponse(template)
return message
} catch(err) {
console.error(err)
}
}
- Create different types of header parameters:
async payload => {
try {
const headerText = new WhatsApp.Header({
type: 'text',
value: 'example text'
}) // type text
const headerMedia = new WhatsApp.Header({
type: 'image', // can be ‘video’ or ‘document’
value: 'https://example.media.com’',
providerName: 'test Provider', //optional
filename: 'test file' //optional
}) // type media
} catch(err) {
console.error(err)
}
}
- Add the parameter to the header:
async payload => {
try {
const header = new WhatsApp.Components({
type: ‘header’
})
const headerText = new WhatsApp.Header({
type:'text',
value: 'example text'
})
header.parameters.push(headerText)
// or
// const headerMedia = new WhatsApp.Header({
// type: 'image',
// value: 'https://example.media.com’',
// providerName: 'test Provider',
// filename: 'test file'
// })
// header.parameters.push( headerMedia )
} catch(err) {
console.error(err)
}
}
- Create different types of body parameters:
async payload => {
try {
const text = new WhatsApp.Header({
"type": "text",
"value": "Example text"
}) // type text
const currency = new WhatsApp.Currency({
"fallback_value": "$10",
"code": "USD",
"amount_1000": 10000 // amount_1000 = actual_value * 1000, here actual_value =10
}) // type currency
const dateTime = new WhatsApp.DateTime({
"fallback_value": "February 29, 2024",
“day_of_week”: 1, // optional
"day_of_month": 25,
"year": 1977,
"month": 2,
"hour": 15,
"minute": 33,
}) // type date_time
} catch(err) {
console.error(err)
}
}
- Add body parameters to the body:
async payload => {
try {
const text = new WhatsApp.Header({
"type": "text",
"value": "Example text"
})
const currency = new WhatsApp.Currency({
"fallback_value": "$10",
"code": "USD",
"amount_1000": 10000
})
const body = new WhatsApp.Components( { type: “body” } )
body.parameters.push(text)
body.parameters.push(currency)
} catch(err) {
console.error(err)
}
}
- Create a template with body and header:
In the example, add the header and body created in the previous steps into the component of the template.
const template = new WhatsApp.Template({
namespace: "your_namespace",
name: "your_template_name",
language: language,
components: [ header, body ] ← add here
})
For more information, read WhatsApp Message Template and Media Message Templates.
Updated about 1 year ago