Code-based Actions: Create a Dynamic URL
You can use a bot to refer to different pages on your website. This is particularly helpful when you have several URLs for different products or features, it reduces the manual effort of building URLs in a dynamic way. To do that within the bot you can use the following three steps:
- Create an entity list
- Create a flow and capture the entity
- Use actions to create a dynamic URL
Create an entity list
Select the Entity types from the menu and create a new list. You can add synonyms and an id as well. Ensure the keyword matches the target link. In the following example, this would be features, solutions, pricing, or docs.
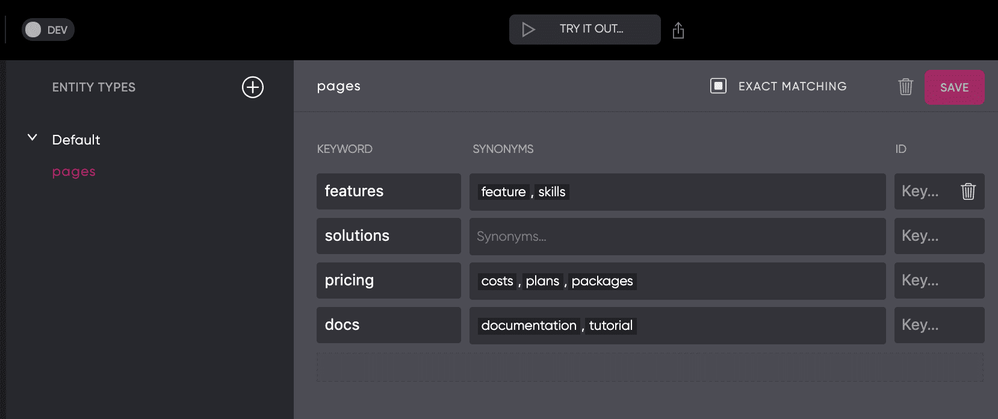
Create a flow and capture the entity
Select the entities and mark them as parameters of the entity list that you have created. In this example, we make the parameter Requiredand must be a valid entry.
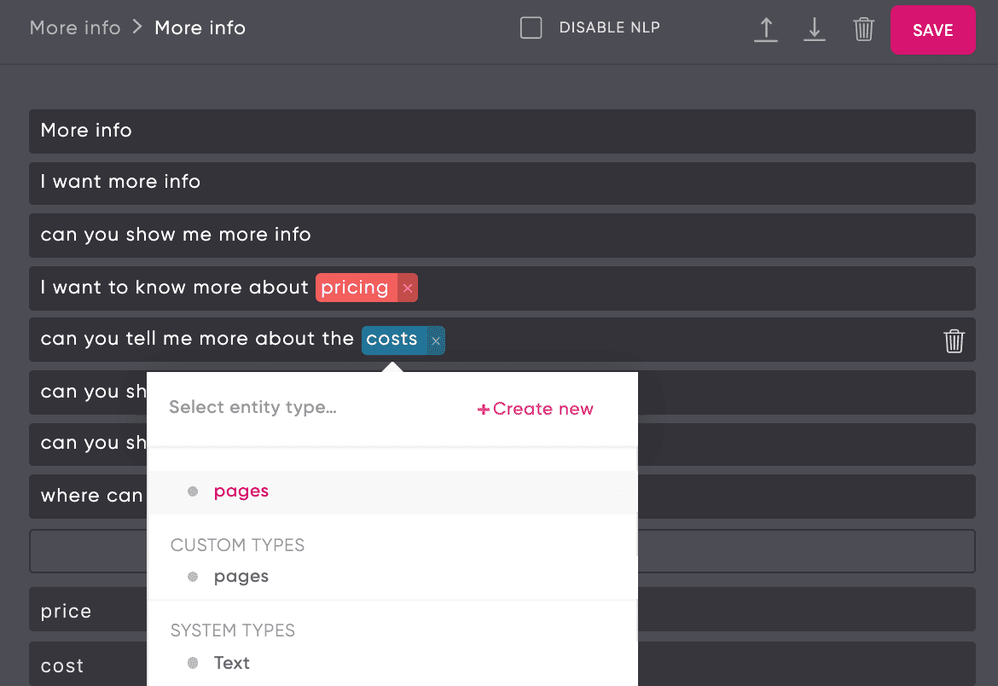
The image below displays that the parameter should be valid and part of the entity list.
Use actions to create a dynamic URL
Rich UI elements such as cards and carousels enhance the customer journey we will create a dynamic url within a card. Let's use two variables. The variable "page" contains the valid value of the captured entity and the variable URL contains the URL that we will be referring to.
async payload => {
const page = payload.params.pages[payload.params.pages.length - 1].value
const URL = `https://flow.ai/${page}`
}
Let's check if we have a value, create a button, and add it to a card to enhance user experience.
if(Array.isArray(payload.params.pages)) {
// Create a Button
const button = new Button({
type: 'url',
label: `More about ${page}`,
value: URL
})
// Create a card
const card = new Card({
title: "Flow.ai Navigation bot",
subtitle: ""
})
.addButton(button)
// Create a message with fallback speech
const message = new Message('More info at Flow.ai')
message.addResponse(card)
return message
}
And we're done!
Find the complete code below:
async payload => {
const page = payload.params.pages[payload.params.pages.length - 1].value
const URL = `https://flow.ai/${page}`
if(Array.isArray(payload.params.pages)) {
// Create a Button
const button = new Button({
type: 'url',
label: `More about ${page}`,
value: URL
})
// Create a card
const card = new Card({
title: "Flow.ai Navigation bot",
subtitle: ""
})
.addButton(button)
// Create a message with fallback speech
const message = new Message('More info at Flow.ai')
message.addResponse(card)
return message
}
}
Updated 5 months ago