Author API example
See a walkthrough example of using the Author API
This guide provides a basic example of using the Author API
to make a bulk update to Khoros Care. The example loads an association between a Twitter ID and a phone number.
Note
Care uses the Twitter ID and not the Twitter handle to uniquely identify
a user. The reason is that a person can change their Twitter handle - it is not a
unique identifier. If you only know the Twitter handle, you can use Twitter's API
to get the ID programmatically, or if you want to get the ID manually, there are a
number of sites that you can use (for example: http://tweeterid.com/).
The code in this example is written in JavaScript for Google's App Script platform. Google docs provide a very convenient way to implement solutions that load data from a spreadsheet - so it is great for this example.
In order to call the Author API, your user must have API access. See user account requirements in HTTP authentication for details.
This example includes the following steps:
- Create a Google spreadsheet
- Add the Google App script
- Use the spreadsheet
Reference script
We'll be using this reference Google App script in the example.
/**
* This function takes in a list of author updates, and call the bulk Author Update API with
* the payload.
*/
function updateLswAuthors(payload) {
var url = "https://abcco.demo.sdxdemo.com" + "/api/v2/authors";
var auth = "Basic " + Utilities.base64Encode("username" + ":" + "password");
var response = UrlFetchApp.fetch(url,
{
method: "put"
,headers: {"Authorization": auth}
,validateHttpsCertificates : false
,payload: JSON.stringify(payload)
});
}
function updateLSW() {
//Get spreadsheet data
//For each 100 rows
// - build the api payload
// - call the api
var spreadSheetData = SpreadsheetApp.getActive().getSheetValues(1,1,SpreadsheetApp.getActive().getLastRow(),2);
var payload = [];
var idx = 1;
do {
var author = { "phone":[], "handles": { "twitter":[] } };
author.phone[0] = String(spreadSheetData[idx][1]);
author.handles.twitter[0] = {"id": spreadSheetData[idx][0]};
payload.push(author);
if (payload.length % 100 == 0 && payload.length > 0) {
updateLswAuthors(payload);
//The next row deletes the entries that were just loaded.
SpreadsheetApp.getActive().deleteRows(2,payload.length);
payload = [];
}
idx += 1;
} while (idx < spreadSheetData.length)
if (payload.length > 0) {
updateLswAuthors(payload);
SpreadsheetApp.getActive().deleteRows(2,payload.length);
}
}
function onOpen() {
var spreadsheet = SpreadsheetApp.getActiveSpreadsheet();
var entries = [{
name : "Update Response",
functionName : "updateLSW"
}];
spreadsheet.addMenu("Social Response Menu", entries);
};
Create a Google spreadsheet
- Log into https://drive.google.com.
- Create a new spreadsheet (and click on the name to rename it).
- Create the input:
a. Add a column for Twitter ID.
b. Add a column for phone number.
The spreadsheet should look something like this:
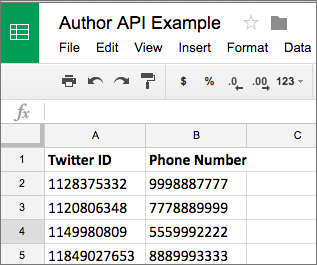
Author API Example - Spredsheet
Add the Google App script
- In the spreadsheet, select Tools > Script Editor.
- Select Blank Project.
- Paste the following code below into the script.
- On Line 7: Replace test.response.lithium.com with your URL.
- On Line 8: Enter your username and password.
- Save the script.
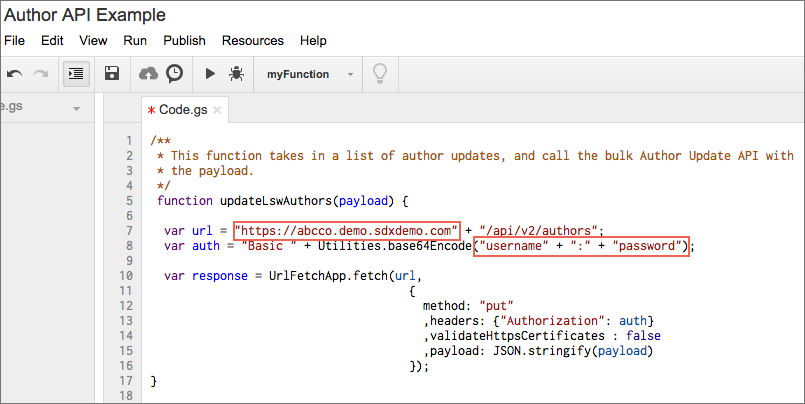
Author API Example - Add the Google App script
Use the spreadsheet
- Close the spreadsheet browser tab (this will also close the script tab).
- Re-open the spreadsheet (in Google Drive, click on the spreadsheet to open it).
- You should now see a menu in the spreadsheet named Social Response Menu with one item Update Response.
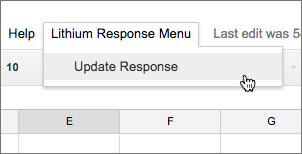
Response Menu
- Enter the data you want to load in the spreadsheet
- Select the Update Response menu item.
Note: the first time you run this, you will have to authorize the script. (Click OK when prompted). - If you look in Care, you should now see these phone numbers associated with the Twitter ID.
Updated almost 5 years ago