iOS Notifications
How to receive push notifications for the chat within your iOS app
Overview
In-app notifications are a crucial way to engage with your users, and in this guide, we'll walk you through the process of setting up notification authorization keys for ios.
How it works is: Brand Messenger sends its payload to Apple servers which then sends the Push notification to your user's device.
There are two types of keys you can use: tokens and certificates. Tokens are the new and preferred way for authentication. If both certificates and tokens are added, tokens will be given preference
Setting up APNs tokens
- Download the .p8 APN auth key file from your Developer Account Help. Click here for information
- You can find your Key ID in the Apple Developer Center under Certificates, Identifiers & Profiles
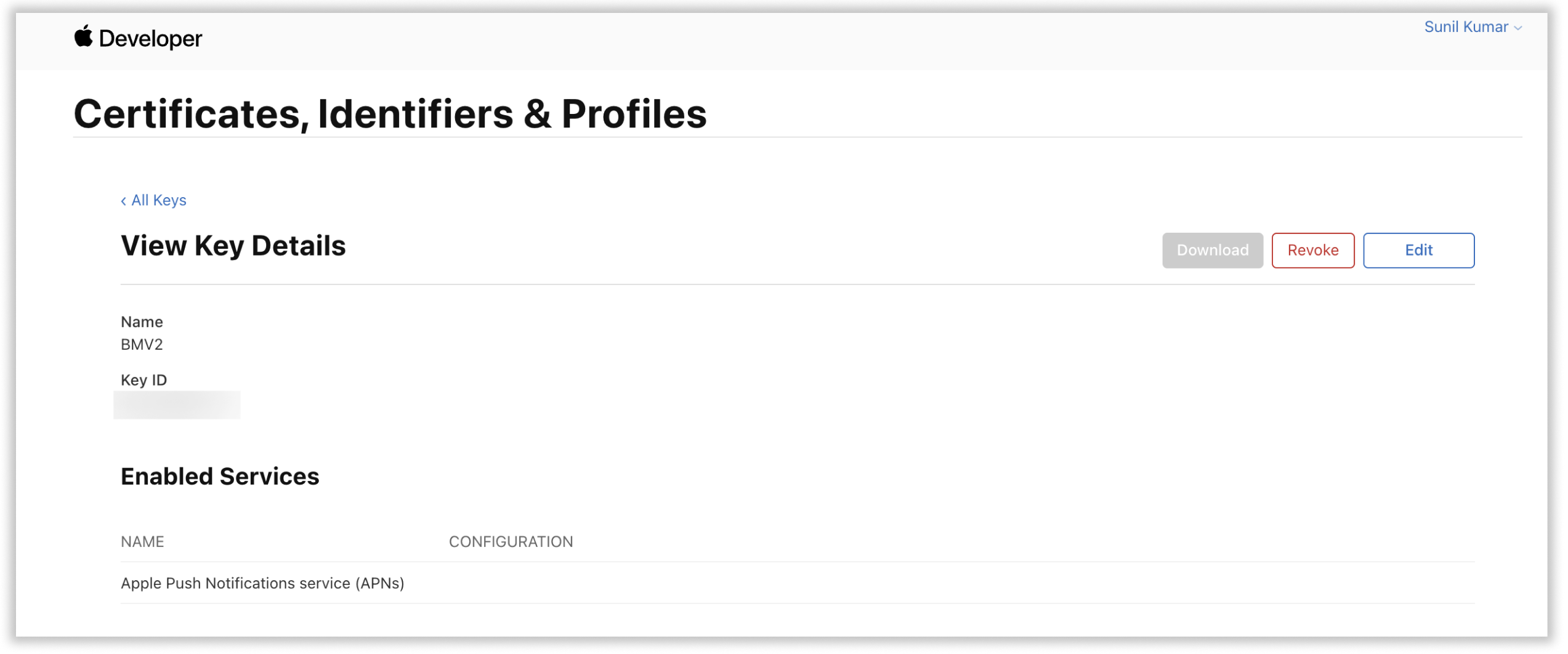
- You can find your Team ID in the Apple Member Center under the Membership details in your Apple Developer account
- In the Apple Developer Center under Identifiers select your app to view Bundle ID
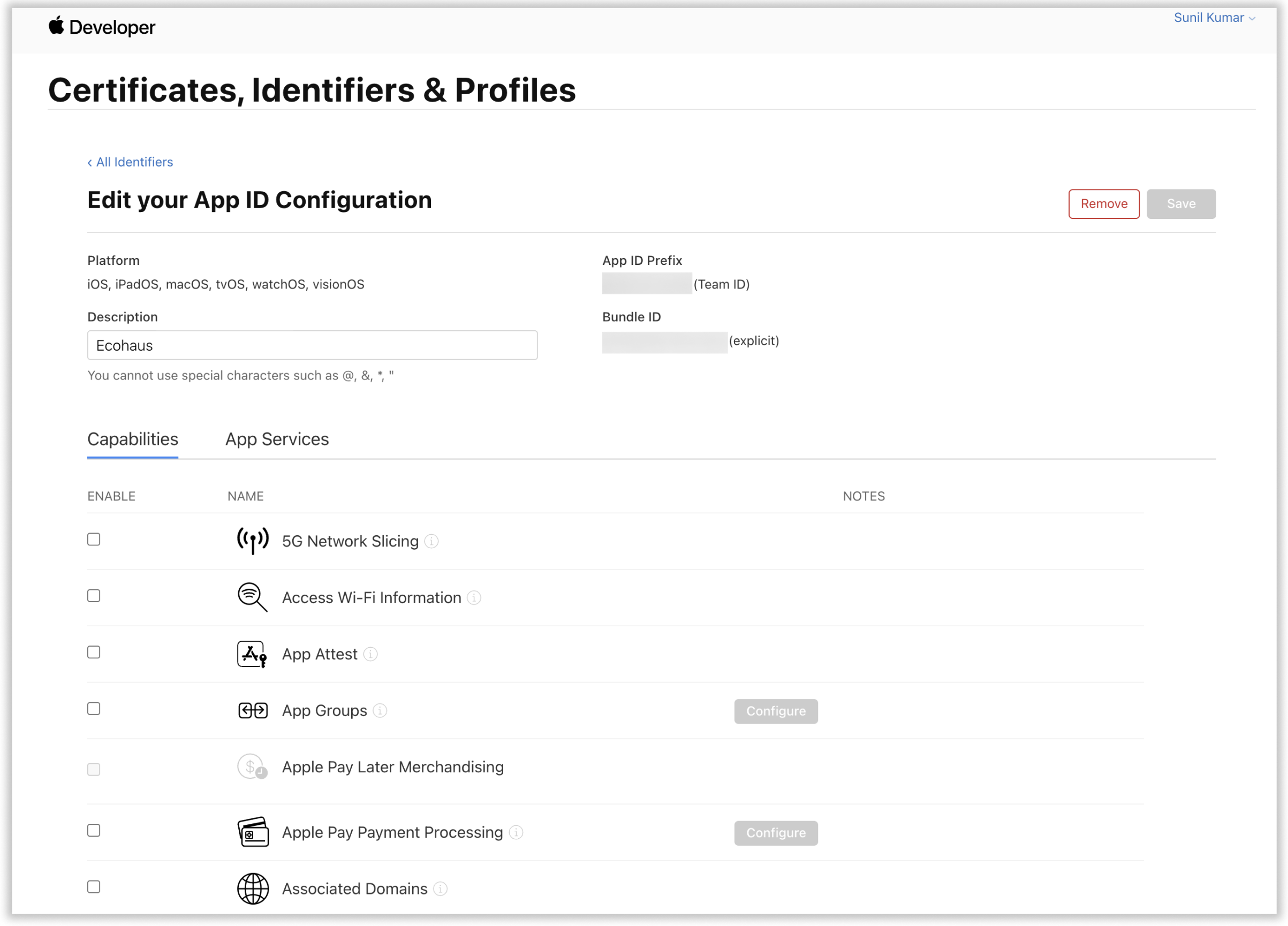
Contact Khoros Support with your .p8 APN auth key file, Key ID, Team ID and Bundle ID to update the APNs keys for your app.
Setting up APNS Certificates
- Visit APNs SSL (Sandbox) to create a development certificate
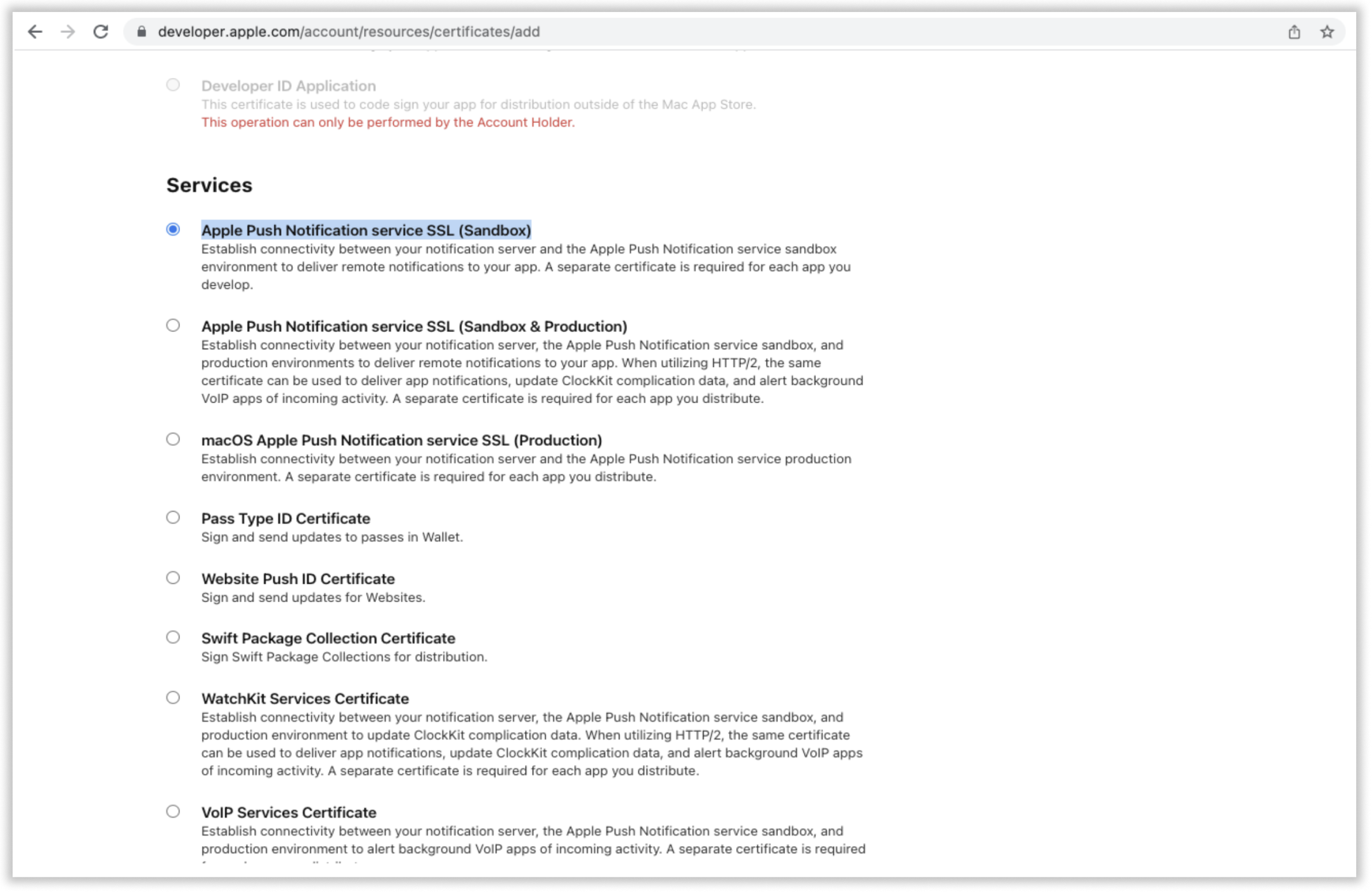
- Visit APNs SSL (Sandbox & Production) to create a development and production certificate
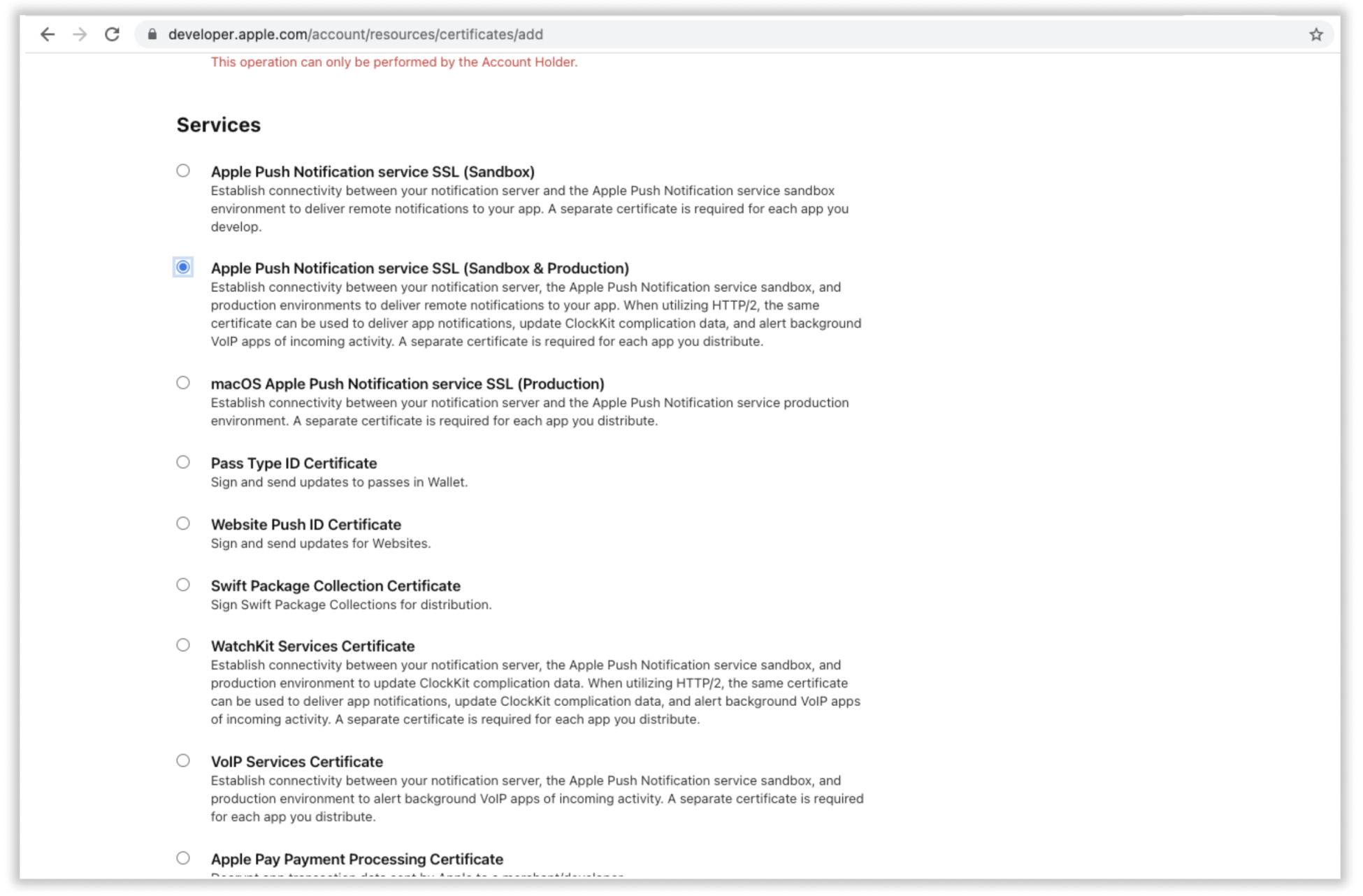
- Download your .p12 certificate for both the environments
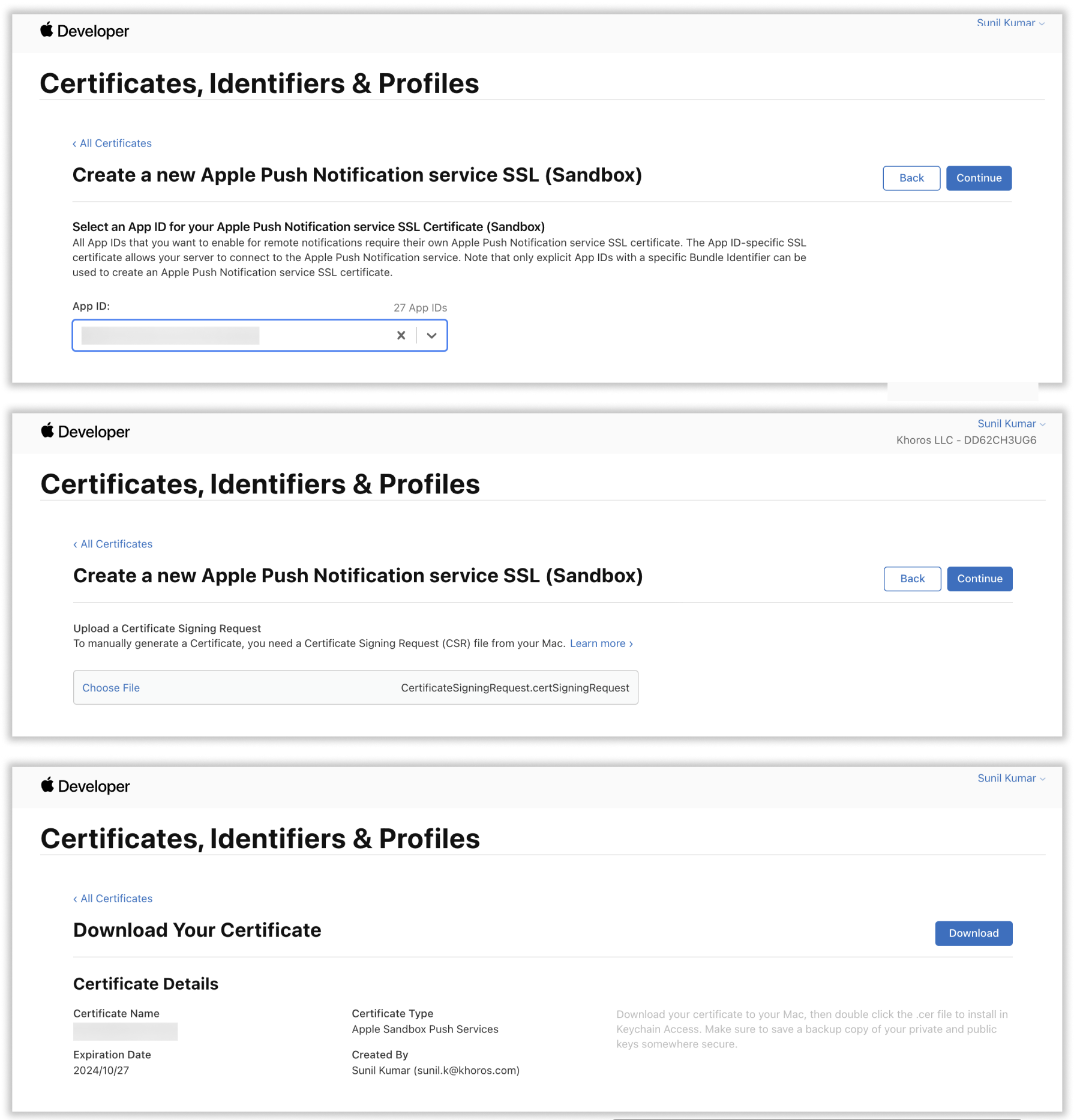
-
Export the .p12 files from keychain
For APNs Production certificate export:
A. Open Keychain Access on your Mac and go to login > Select My Certificates.
B. Find the Apple push services certificate you added, right-click on the certificate, and select the Export option from the menu to get a .p12 file. Type a name for the file and save it with the password.
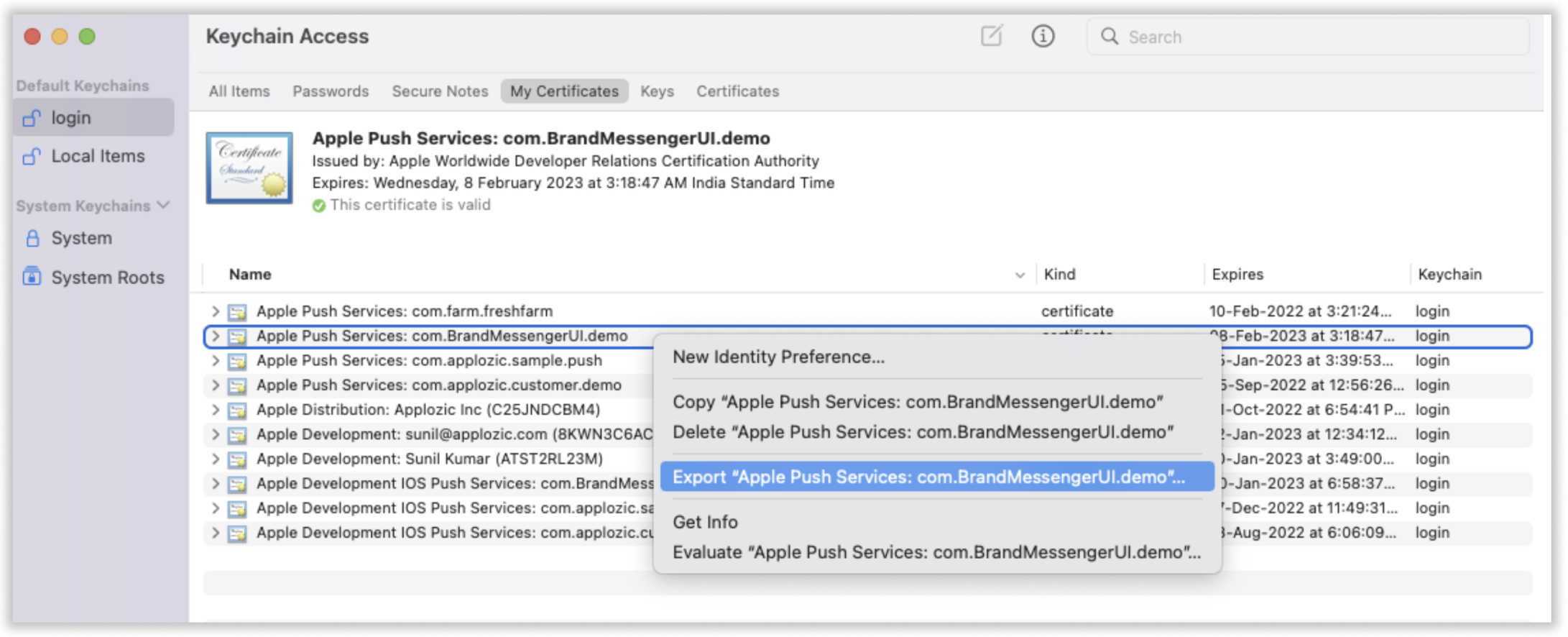
For APNS sandbox certificate export :
A. Open Keychain Access on your Mac and go to login > Select My Certificates.
B. Find the APNS Development iOS certificate you added, right-click on the certificate, and select the Export option from the menu to get a .p12 file. Type a name for the file and save it with the password.
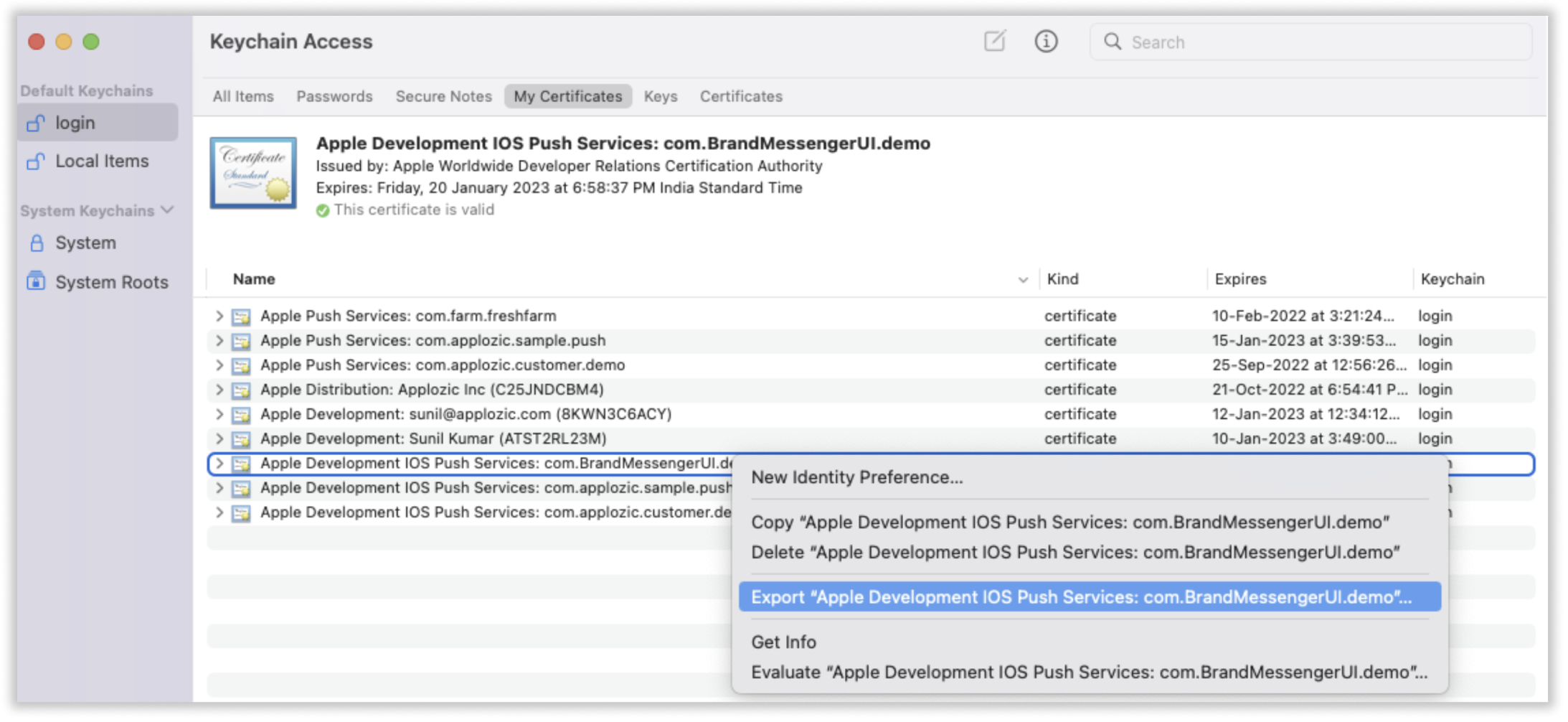
Please reach out to Khoros Support team and share your exported .p12 files for both environments, along with the corresponding passwords, to facilitate the upload of your push notification certificates.
Updating Capabilities
After setting up the APNS, you must enable push notifications within your project.
Click on your project, click Capabilities, and in Background Modes, enable Background Fetch and Remote notifications
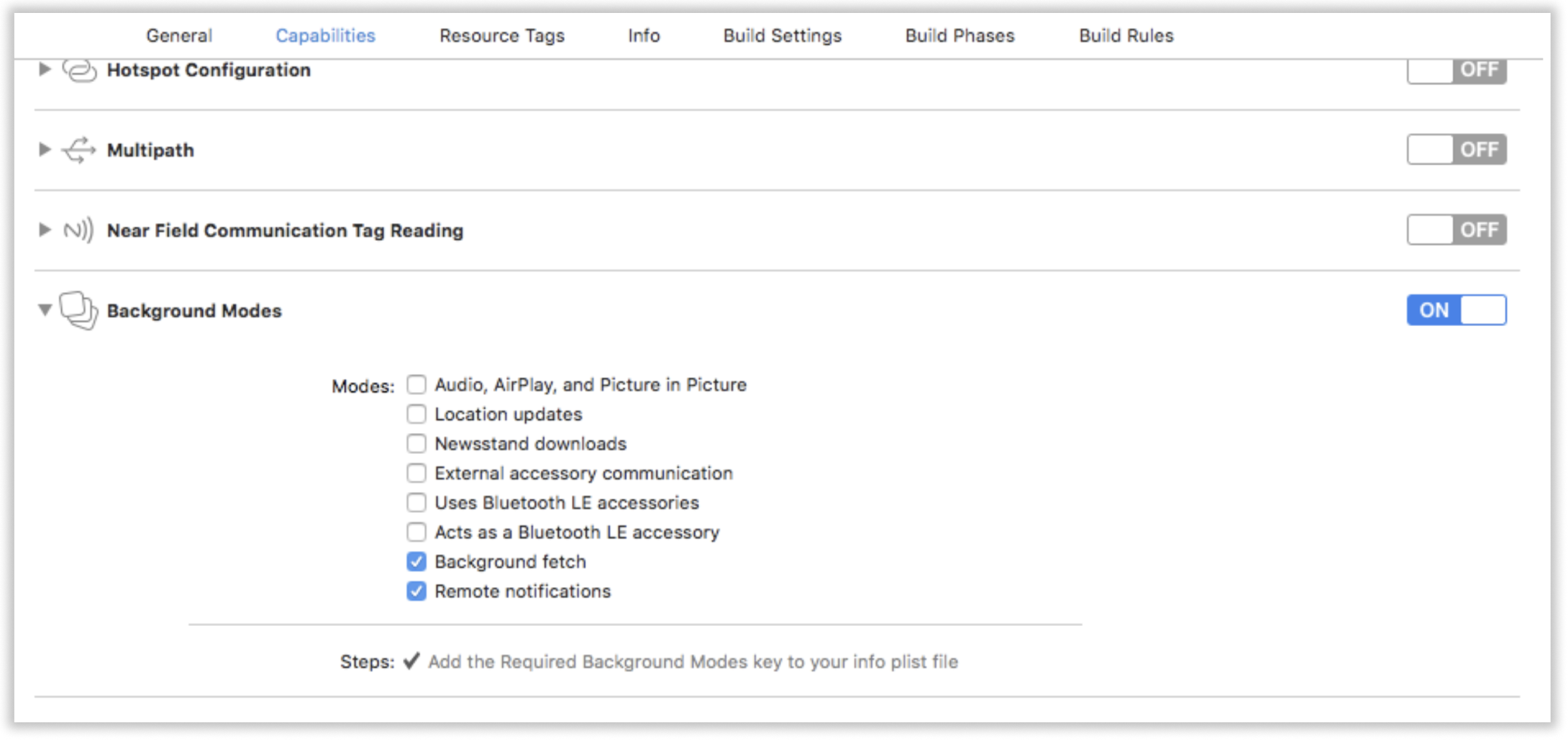
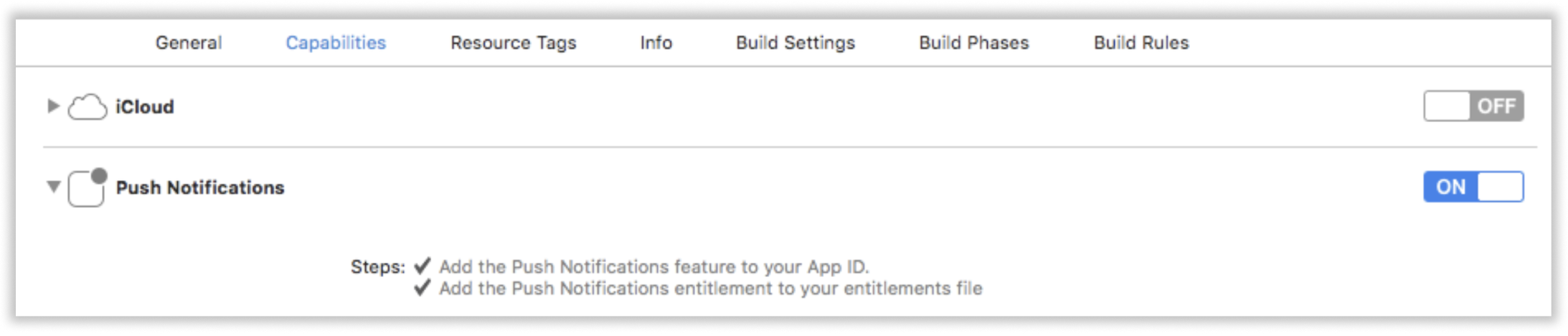
Setup Push Notifications in-app
Push notification setup is rather straightforward, all you need to do is to find the AppDelegate file in your project and follow all the instructions below.
To enable push notifications, insert the following code into your AppDelegate.swift or AppDelegate.m file.
If the method below already exists in your file, simply copy the code within the method and paste it into your AppDelegate file.
Make sure your AppDelegate conforms to UNUserNotificationCenterDelegate and make sure that each of the methods or code inside the method is added in your Appdelegate file
Sample code is as below:
import BrandMessengerUI
import UIKit
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate, UNUserNotificationCenterDelegate {
var window: UIWindow?
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
// Push notification register.
UNUserNotificationCenter.current().delegate = self
BrandMessengerManager.application(application, didFinishLaunchingWithOptions: launchOptions)
return true
}
func applicationWillEnterForeground(_ application: UIApplication) {
// Reset the app badge count
BrandMessengerManager.applicationWillEnterForeground(application)
}
func applicationWillTerminate(_ application: UIApplication) {
// Save context in app will Terminate
BrandMessengerManager.applicationWillTerminate(application: application)
}
func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) {
// Register device token to Brand Messenger server.
BrandMessengerManager.application(application, didRegisterForRemoteNotificationsWithDeviceToken: deviceToken)
}
func application(_ application: UIApplication, didReceiveRemoteNotification userInfo: [AnyHashable : Any], fetchCompletionHandler completionHandler: @escaping (UIBackgroundFetchResult) -> Void) {
// Pass the notification to the Brand Messenger method for processing.
BrandMessengerManager.application(application, didReceiveRemoteNotification: userInfo) { result in
// Process your own notification here.
completionHandler(result)
}
}
func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
// Pass the notification to the Brand Messenger method for processing.
BrandMessengerManager.userNotificationCenter(center, willPresent: notification) { options in
// Process your own notification here.
completionHandler(options)
}
}
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
// Pass the response to the Brand Messenger method for processing notification.
BrandMessengerManager.userNotificationCenter(center, didReceive: response) {
// Process your own notification here.
completionHandler()
}
}
}
#import "AppDelegate.h"
@import BrandMessengerCore;
#import "YOUR_TARGET_NAME_HERE-Swift.h" // Important: Replace 'YOUR_TARGET_NAME_HERE' with your target name.
#import <UserNotifications/UserNotifications.h>
/// Add this UNUserNotificationCenterDelegate in your Appdelegate file
@interface AppDelegate () <UNUserNotificationCenterDelegate>
@end
@implementation AppDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
// Push notification register.
UNUserNotificationCenter.currentNotificationCenter.delegate = self;
[BrandMessengerManager application:application didFinishLaunchingWithOptions:launchOptions];
return YES;
}
-(void)application:(UIApplication *)application
didReceiveRemoteNotification:(NSDictionary *)userInfo
fetchCompletionHandler:(nonnull void (^)(UIBackgroundFetchResult))completionHandler {
[BrandMessengerManager application:application didReceiveRemoteNotification:userInfo fetchCompletionHandler:^(UIBackgroundFetchResult backgroundFetchResult) {
// Perform your other operations here
completionHandler(backgroundFetchResult);
}];
}
- (void)userNotificationCenter:(UNUserNotificationCenter *)center willPresentNotification:(UNNotification*)notification
withCompletionHandler:(void (^)(UNNotificationPresentationOptions options))completionHandler {
[BrandMessengerManager userNotificationCenter:center willPresent:notification withCompletionHandler:^(UNNotificationPresentationOptions options) {
// Process your own notification here.
completionHandler(options);
}];
}
- (void)userNotificationCenter:(UNUserNotificationCenter *)center
didReceiveNotificationResponse:(nonnull UNNotificationResponse* )response
withCompletionHandler:(nonnull void (^)(void))completionHandler {
[BrandMessengerManager userNotificationCenter:center didReceive:response withCompletionHandler:^{
// Process your own notification here.
completionHandler();
}];
}
- (void)applicationWillEnterForeground:(UIApplication *)application {
[BrandMessengerManager applicationWillEnterForeground:application];
}
- (void)applicationWillTerminate:(UIApplication *)application {
[BrandMessengerManager applicationWillTerminateWithApplication:application];
}
- (void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken {
[BrandMessengerManager application:application didRegisterForRemoteNotificationsWithDeviceToken:deviceToken];
}
@end
Note: The code provided above in the didFinishLaunchingWithOptions for BrandMessengerManager is mandatory in all scenarios. If you are utilising a different push notification setup, such as Firebase or OneSignal, it must also be included in your AppDelegate file.
Customising alert sounds
In order to disable the incoming push notification alert or only notification sound, you need to update notification mode. Below are the possible values of notification mode.
Notification Mode | Explanation |
---|---|
0 | Enable notification with sound (Default) |
1 | Enable notification without sound |
2 | Disable notification (No Alert) |
KBMRegisterUserClientService.updateNotificationMode(modeValue, withCompletion: {
response, error in
if(error != nil) {
// Failed to update notification mode
} else {
KBMUserDefaultsHandler.setNotificationMode(modeValue)
}
})
Customising Push Notifications
When using generic push-notifications, you can customise the title and text of the incoming push-notification displayed in the iOS Banner in the following way
- Create new Notification Service Extension target
In Xcode, within your project's TARGETS
, include a new target of the Notification Service Extension
type. You can find official instructions here.
This will generate a new NotificationService
file.
- Update NotificationService file
import UserNotifications
class NotificationService: UNNotificationServiceExtension {
var contentHandler: ((UNNotificationContent) -> Void)?
var bestAttemptContent: UNMutableNotificationContent?
override func didReceive(_ request: UNNotificationRequest, withContentHandler contentHandler: @escaping (UNNotificationContent) -> Void) {
self.contentHandler = contentHandler
bestAttemptContent = (request.content.mutableCopy() as? UNMutableNotificationContent)
if let bestAttemptContent = bestAttemptContent {
// Modify the notification content here...
/// Brand messenger notification
if isBrandMessengerChatNotificaiton(content: request.content) {
bestAttemptContent.title = NSLocalizedString("GenericNotificationTitle", tableName: nil, bundle: .main, value: "Chat", comment: "")
bestAttemptContent.body = NSLocalizedString("GenericNotificationContentText", tableName: nil, bundle: .main, value: "You have a new message.", comment: "")
}
contentHandler(bestAttemptContent)
}
}
override func serviceExtensionTimeWillExpire() {
// Called just before the extension will be terminated by the system.
// Use this as an opportunity to deliver your "best attempt" at modified content, otherwise the original push payload will be used.
if let contentHandler = contentHandler, let bestAttemptContent = bestAttemptContent {
contentHandler(bestAttemptContent)
}
}
/// Use this method to check for notification for Brand Messenger.
/// - Parameter content:Pass `UNNotificationContent` object for notification userInfo payload.
/// - Returns: Returns `true` if it's a Brand Messenger notification otherwise `false`.
func isBrandMessengerChatNotificaiton(content: UNNotificationContent) -> Bool {
let prefix = "BRANDMESSENGER_"
let notificationKey = "AL_KEY"
guard let brandMessengerPrefix = content.userInfo[notificationKey] as? String, brandMessengerPrefix.hasPrefix(prefix) else {
return false
}
return true
}
}
- Bundle Localizable.strings into the created extension
In Xcode, in Project settings, select the newly created Notification Service Extension
TARGET
.
Go to Build Phases
tab, and in Copy Bundle Resources
, add the app's Localizable.strings
file. If one does not already exist, create one and add.
- Modify the notification strings
In the Localizable.strings
file, add values for GenericNotificationTitle
and GenericNotificationContentText
to set them on the incoming push-notification banners.
GenericNotificationTitle = "Khoros Chat";
GenericNotificationContentText = "You have a new message!";
Verifying a Brand Messenger notification
You can verify whether it's a Brand Messenger notification with the following code:
NSDictionary * userInfo = response.notification.request.content.userInfo;
KBMPushNotificationService *service = [[KBMPushNotificationService alloc]init];
if ([service isBrandMessengerChatNotification: userInfo]) {
// Brand Messenger notification
} else {
//Handle your notification
}
}
Updated 7 months ago